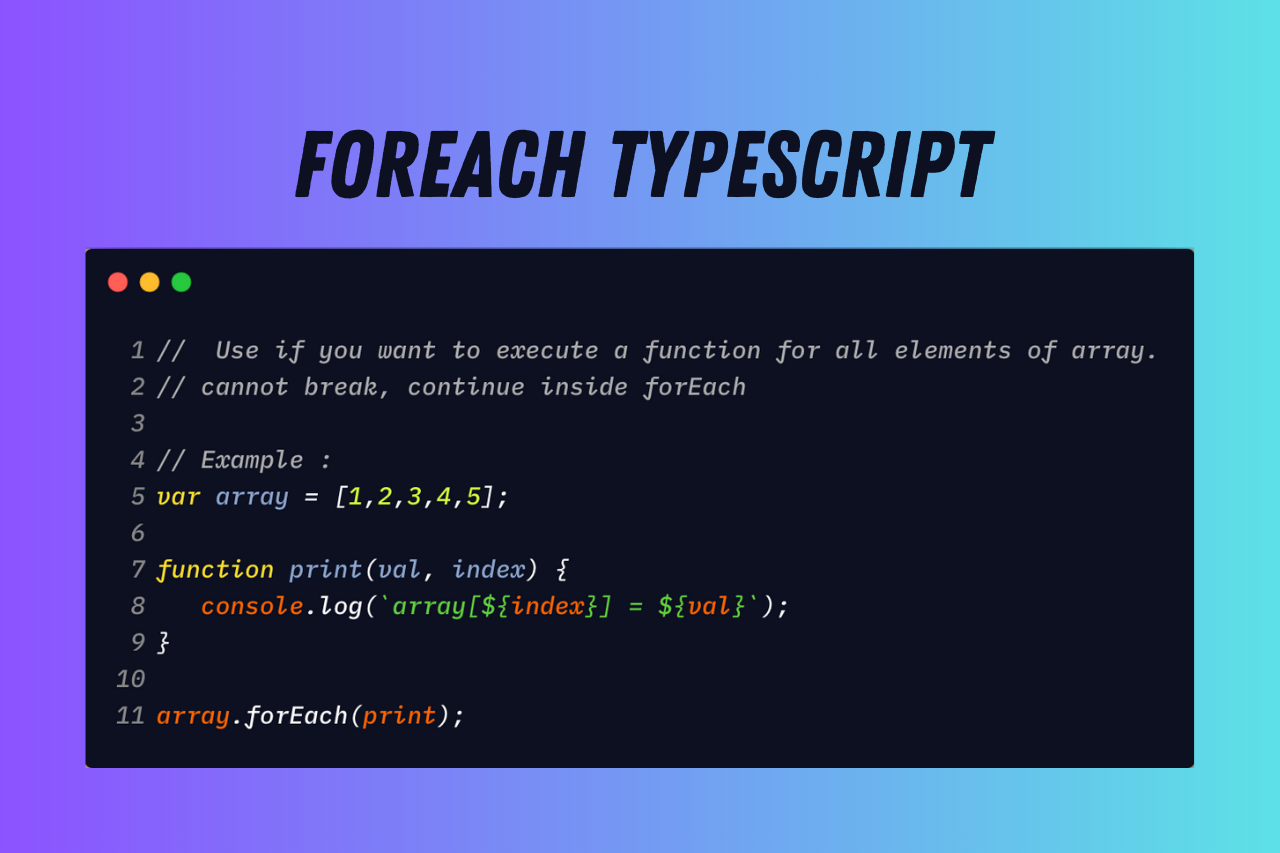
foreach typescript
In the world of programming, efficient iteration through collections of data is a crucial aspect of writing clean and concise code. TypeScript, a superset of JavaScript, offers developers various tools to handle iterations effectively. One such tool is the foreach loop, which simplifies the process of iterating through arrays, objects, and custom data types. In this article, we will delve into the intricacies of foreach typescript in TypeScript, exploring its syntax, applications, advantages, and limitations.
Introduction to foreach loop
Before diving into TypeScript specifics, let’s briefly revisit the concept of the foreach loop. In general programming terminology, a foreach loop is a control flow statement used for iterating over a collection of items. It iterates over each item in the collection and executes a block of code for each iteration.
Basics of TypeScript
TypeScript is an open-source programming language created and kept up with by Microsoft. It is a statically typed language that builds on the syntax and features of JavaScript, adding optional static typing and other advanced features. TypeScript is widely used for developing large-scale applications due to its ability to catch errors early in the development process and improve code maintainability.
What is foreach in TypeScript?
In TypeScript, the foreach typescript loop provides a convenient way to iterate over the elements of an array, the properties of an object, or the elements of a custom data type. It offers a cleaner and more readable alternative to traditional loops, especially when dealing with complex data structures.
Syntax of foreach loop in TypeScript
The syntax of the foreach loop in TypeScript is easy to understand. It follows the pattern:
forEach(element: T, index: number, array: T[]) => void
Where:
- component: Addresses the ongoing component being handled in the cluster.
- index: Represents the index of the current element being processed.
- array: Represents the array that foreach typescript is being applied to.
Understanding the iteration process
When using foreach in TypeScript, the loop iterates over each element of the array, object, or custom data type, executing the provided callback function for each iteration. This allows developers to perform operations on each element without explicitly managing loop counters or indices.
Advantages of using foreach loop
The foreach loop offers several advantages over traditional for loops, including:
- Simplified syntax: The syntax of foreach typescript is more concise and readable than traditional loops.
- Automatic iteration: Developers don’t need to manage loop counters or indices manually.
- Enhanced code readability: foreach loops make the intent of the code clearer, improving overall code readability.
Limitations of foreach loop
Despite its advantages, the foreach loop has some limitations:
- Limited control: foreach loops offer less control over the iteration process compared to traditional loops.
- Cannot break or continue: Unlike for loops, foreach typescript loops cannot be terminated prematurely using break or continue statements.
Foreach typescript
In TypeScript, the “foreach” loop, also known as the “for…of” loop, is a powerful construct used to iterate over elements of an array, collection, or iterable object. Unlike traditional loops, which require manual indexing and iteration, the foreach loop simplifies the process by automatically iterating over each element of the array or iterable object. This makes code more concise and readable, especially when dealing with complex data structures. Additionally, the foreach loop supports the use of iterators, allowing for seamless integration with various data types and custom data structures. Overall, the foreach loop in TypeScript enhances code efficiency and readability, making it a valuable tool for developers.
Practical examples of foreach loop in TypeScript
Let’s explore some practical examples of using the foreach typescript loop in TypeScript:
Using foreach with arrays
let numbers: number[] = [1, 2, 3, 4, 5];
numbers.forEach((num) => {
console.log(num);
});
Using foreach with objects
let person = { name: ‘John’, age: 30, city: ‘New York’ };
Object.keys(person).forEach((key) => {
console.log(`${key}: ${person[key]}`);
});
Using foreach with custom data types
class Employee {
constructor(public name: string, public job: string) {}
}
let employees: Employee[] = [
new Employee(‘Alice’, ‘Manager’),
new Employee(‘Bob’, ‘Developer’),
new Employee(‘Charlie’, ‘Designer’)
];
employees.forEach((emp) => {
console.log(`${emp.name} – ${emp.role}`);
});
Tips for effective use of foreach loop
To make the most out of foreach typescript loops in TypeScript, consider the following tips:
- Ensure clarity: Use descriptive variable names and meaningful callback functions to enhance code clarity.
- Handle asynchronous operations: If the callback function contains asynchronous code, ensure proper error handling and asynchronous control flow.
- Avoid modifying the collection: Avoid modifying the array, object, or custom data type being iterated over within the loop to prevent unexpected behavior.
Comparing foreach with other iteration methods
While foreach offers simplicity and readability, it’s essential to understand its differences from other iteration methods, such as for loops, maps, filters, and reduce. Each method has its strengths and weaknesses, and choosing the right one depends on the specific requirements of the task at hand.
Best practices for writing foreach loops
To write efficient and maintainable foreach typescript loops in TypeScript, follow these best practices:
- Use type annotations: Specify the type of elements in the array or object to enable type checking and improve code robustness.
- Optimize performance: Avoid unnecessary computations or operations within the loop to optimize performance, especially when dealing with large datasets.
- Test thoroughly: Test foreach typescript loops with different input scenarios to ensure they behave as expected and handle edge cases gracefully.
Conclusion
In conclusion, a foreach typescript loop is a powerful tool in the TypeScript developer’s arsenal for iterating over collections of data efficiently. By understanding its syntax, applications, advantages, and limitations, developers can leverage foreach loops to write cleaner, more concise, and more maintainable code.
FAQs
1. Can I use foreach with asynchronous operations in TypeScript?
Yes, you can use foreach typescript with asynchronous operations by handling promises or using async/await syntax within the callback function.
2. Is the foreach loop supported in all versions of TypeScript?
Yes, the foreach typescript loop is supported in all versions of TypeScript since it is a standard feature inherited from JavaScript.
3. How does foreach differ from other iteration methods like map and filter?
Unlike map and filter, which return new arrays, foreach typescript is used for executing a callback function on each element of the array without creating a new array.
4. Can I break out of a foreach loop in TypeScript?
No, you cannot break out of a foreach typescript loop using a break statement. If you need to terminate the loop prematurely, consider using a traditional for loop instead.
5. Are foreach loops recommended for performance-critical applications?
While foreach typescript loops offer simplicity and readability, they may not be the most performant option for handling large datasets or performance-critical applications. In such cases, consider using other iteration methods or optimizing the loop for better performance.